How can I use JavaScript to print real-time cryptocurrency prices on my website?

I want to display real-time cryptocurrency prices on my website using JavaScript. How can I achieve this?
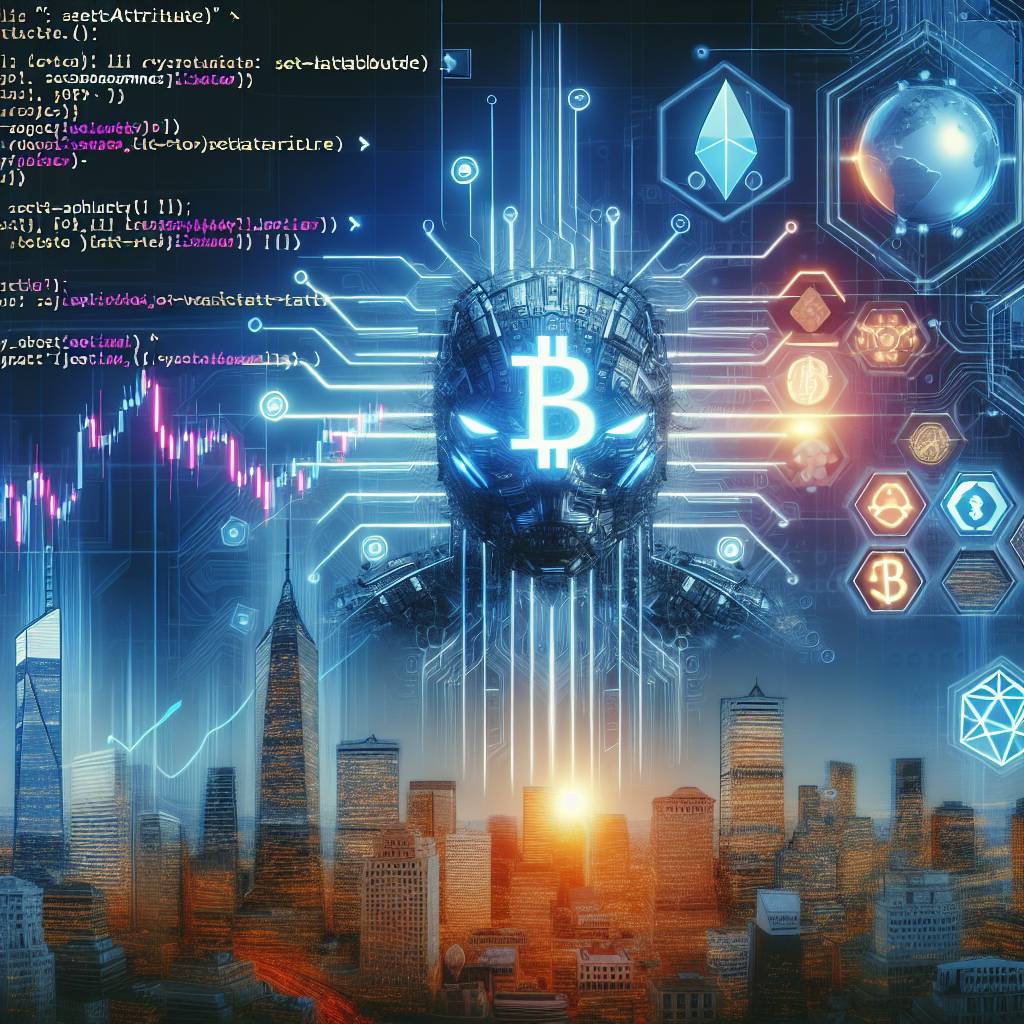
3 answers
- One way to print real-time cryptocurrency prices on your website using JavaScript is by utilizing an API. There are several cryptocurrency APIs available that provide real-time price data. You can make an HTTP request to the API endpoint and retrieve the data in JSON format. Then, you can parse the JSON response and extract the relevant price information. Finally, you can use JavaScript to dynamically update the price on your website. For example, you can use the CoinGecko API to get real-time cryptocurrency prices. Here's a sample code snippet: ```javascript fetch('https://api.coingecko.com/api/v3/simple/price?ids=bitcoin&vs_currencies=usd') .then(response => response.json()) .then(data => { const bitcoinPrice = data.bitcoin.usd; document.getElementById('price').innerText = bitcoinPrice; }); ``` In this code, we fetch the price of Bitcoin in USD from the CoinGecko API and update the element with the id 'price' on our website with the retrieved price.
Dec 16, 2021 · 3 years ago
- To print real-time cryptocurrency prices on your website using JavaScript, you can also consider using a JavaScript library like WebSocket. WebSocket allows for real-time communication between the client and the server. You can establish a WebSocket connection with a cryptocurrency exchange or a data provider that offers real-time price updates. Once the connection is established, you can receive real-time price data and update your website accordingly. Here's an example code snippet using the WebSocket API: ```javascript const socket = new WebSocket('wss://api.example.com'); socket.onmessage = function(event) { const data = JSON.parse(event.data); const bitcoinPrice = data.bitcoin.usd; document.getElementById('price').innerText = bitcoinPrice; }; ``` In this code, we establish a WebSocket connection with the server and listen for incoming messages. When a new message is received, we parse the JSON data and update the element with the id 'price' on our website with the Bitcoin price in USD.
Dec 16, 2021 · 3 years ago
- At BYDFi, we provide a simple JavaScript widget that allows you to display real-time cryptocurrency prices on your website. You can easily integrate the widget by adding a few lines of code to your website. The widget supports various customization options, such as choosing the cryptocurrencies to display, the display format, and the color scheme. Here's an example code snippet to integrate the BYDFi widget: ```html <script src="https://widget.bydfi.com/widget.js"></script> <div id="bydfi-widget"></div> <script> BYDFiWidget.init({ apiKey: 'YOUR_API_KEY', cryptocurrencies: ['bitcoin', 'ethereum'], format: 'price', theme: 'light' }); </script> ``` In this code, you need to replace 'YOUR_API_KEY' with your actual API key from BYDFi. You can also customize the cryptocurrencies, format, and theme according to your preferences. The BYDFi widget will then display the real-time prices of the specified cryptocurrencies on your website.
Dec 16, 2021 · 3 years ago
Related Tags
Hot Questions
- 98
What are the tax implications of using cryptocurrency?
- 95
Are there any special tax rules for crypto investors?
- 93
What is the future of blockchain technology?
- 75
What are the advantages of using cryptocurrency for online transactions?
- 67
How can I buy Bitcoin with a credit card?
- 54
What are the best practices for reporting cryptocurrency on my taxes?
- 52
How can I minimize my tax liability when dealing with cryptocurrencies?
- 47
How can I protect my digital assets from hackers?