How can I use JavaScript to sort a list of digital currencies based on market capitalization?

I want to create a JavaScript function that can sort a list of digital currencies based on their market capitalization. How can I achieve this? Are there any specific libraries or APIs that can help with this task?
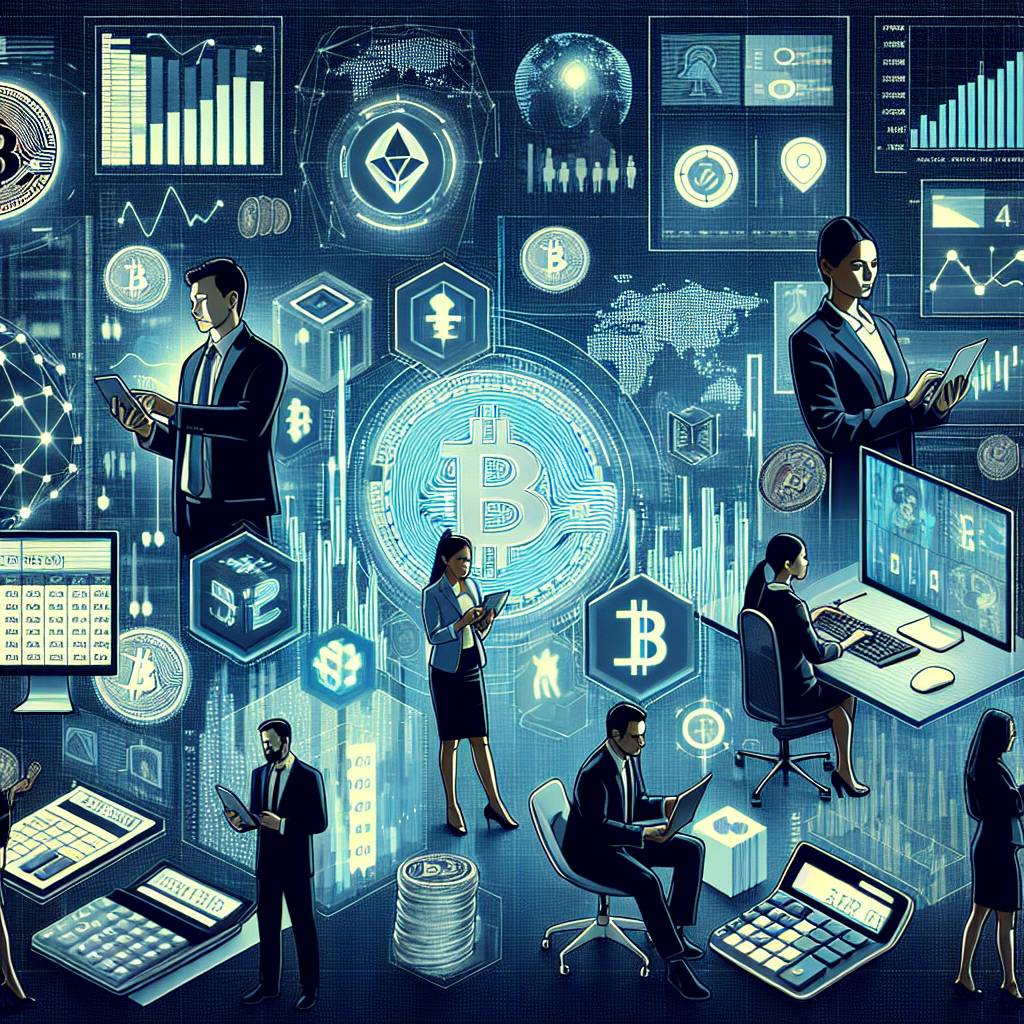
3 answers
- Sure, you can use JavaScript's built-in array sorting method to achieve this. First, you need to have an array of objects, where each object represents a digital currency and has a 'marketCap' property. Then, you can use the 'sort' method with a custom compare function that compares the 'marketCap' property of two objects. This will sort the array in ascending order of market capitalization. Here's an example code snippet: ```javascript const currencies = [ { name: 'Bitcoin', marketCap: 1000000000 }, { name: 'Ethereum', marketCap: 500000000 }, { name: 'Ripple', marketCap: 250000000 } ]; function compareByMarketCap(a, b) { return a.marketCap - b.marketCap; } currencies.sort(compareByMarketCap); console.log(currencies); ``` This will output the sorted array of currencies based on their market capitalization.
Dec 17, 2021 · 3 years ago
- Sorting a list of digital currencies based on market capitalization using JavaScript is a common task in the world of cryptocurrency. One popular library that can help with this is 'lodash'. Lodash provides a variety of utility functions, including sorting arrays of objects based on a specific property. You can use the 'sortBy' function from lodash to sort your list of digital currencies based on their market capitalization. Here's an example code snippet: ```javascript const _ = require('lodash'); const currencies = [ { name: 'Bitcoin', marketCap: 1000000000 }, { name: 'Ethereum', marketCap: 500000000 }, { name: 'Ripple', marketCap: 250000000 } ]; const sortedCurrencies = _.sortBy(currencies, 'marketCap'); console.log(sortedCurrencies); ``` This will output the sorted array of currencies based on their market capitalization using lodash.
Dec 17, 2021 · 3 years ago
- BYDFi is a digital currency exchange that offers a wide range of trading options. While I cannot specifically recommend BYDFi for this task, you can explore their platform and see if they provide any APIs or libraries that can help with sorting digital currencies based on market capitalization. It's always a good idea to research and compare different options before making a decision.
Dec 17, 2021 · 3 years ago
Related Tags
Hot Questions
- 98
What are the best digital currencies to invest in right now?
- 87
What is the future of blockchain technology?
- 83
What are the advantages of using cryptocurrency for online transactions?
- 73
What are the tax implications of using cryptocurrency?
- 54
How can I minimize my tax liability when dealing with cryptocurrencies?
- 44
What are the best practices for reporting cryptocurrency on my taxes?
- 39
How can I buy Bitcoin with a credit card?
- 37
Are there any special tax rules for crypto investors?