How can regular expressions be used to validate cryptocurrency wallet addresses in JavaScript?

Can you provide an example of how regular expressions can be used to validate cryptocurrency wallet addresses in JavaScript?
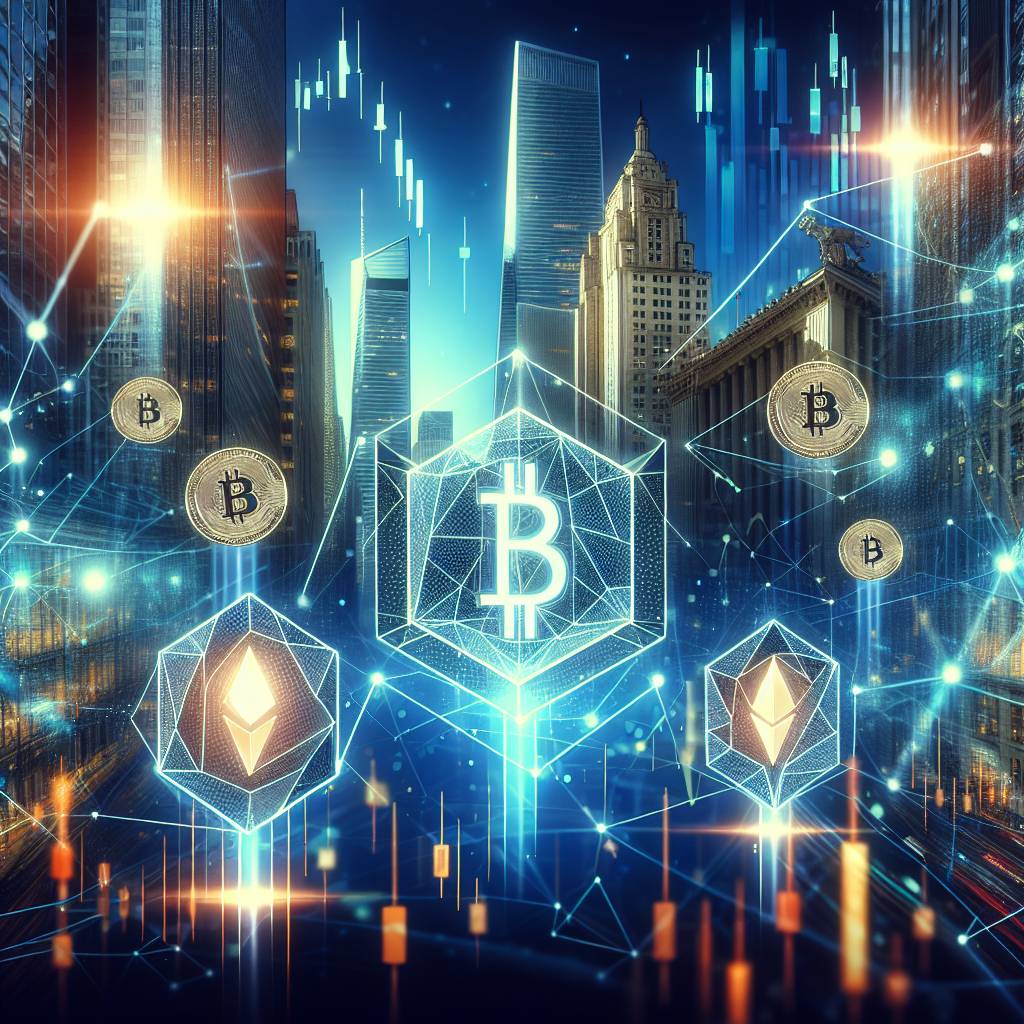
3 answers
- Sure! Regular expressions are a powerful tool in JavaScript that can be used to validate cryptocurrency wallet addresses. Here's an example of how you can use a regular expression to validate a Bitcoin wallet address: ```javascript function validateBitcoinAddress(address) { const bitcoinRegex = /^[13][a-km-zA-HJ-NP-Z1-9]{25,34}$/; return bitcoinRegex.test(address); } const addressToValidate = '1BvBMSEYstWetqTFn5Au4m4GFg7xJaNVN2'; console.log(validateBitcoinAddress(addressToValidate)); // Output: true ``` In this example, the regular expression `/^[13][a-km-zA-HJ-NP-Z1-9]{25,34}$/` is used to validate a Bitcoin wallet address. It checks if the address starts with either 1 or 3, followed by a combination of letters and numbers with a length between 25 and 34 characters. The `test()` method is then used to check if the address matches the regular expression. Remember to adjust the regular expression based on the specific requirements of the cryptocurrency you are validating.
Nov 27, 2021 · 3 years ago
- Definitely! Regular expressions are a handy tool for validating cryptocurrency wallet addresses in JavaScript. Here's an example of how you can use a regular expression to validate an Ethereum wallet address: ```javascript function validateEthereumAddress(address) { const ethereumRegex = /^0x[a-fA-F0-9]{40}$/; return ethereumRegex.test(address); } const addressToValidate = '0x742d35Cc6634C0532925a3b844Bc454e4438f44e'; console.log(validateEthereumAddress(addressToValidate)); // Output: true ``` In this example, the regular expression `/^0x[a-fA-F0-9]{40}$/` is used to validate an Ethereum wallet address. It checks if the address starts with '0x' followed by 40 hexadecimal characters. The `test()` method is then used to check if the address matches the regular expression. Remember to adjust the regular expression based on the specific requirements of the cryptocurrency you are validating.
Nov 27, 2021 · 3 years ago
- Yes, regular expressions can be used to validate cryptocurrency wallet addresses in JavaScript. Here's an example of how you can use a regular expression to validate a Litecoin wallet address: ```javascript function validateLitecoinAddress(address) { const litecoinRegex = /^[LM3][a-km-zA-HJ-NP-Z1-9]{26,33}$/; return litecoinRegex.test(address); } const addressToValidate = 'LbYp8vB7rUJ2c7QbDn6U1V5a7hJ6Cz3J8K'; console.log(validateLitecoinAddress(addressToValidate)); // Output: true ``` In this example, the regular expression `/^[LM3][a-km-zA-HJ-NP-Z1-9]{26,33}$/` is used to validate a Litecoin wallet address. It checks if the address starts with either L, M, or 3, followed by a combination of letters and numbers with a length between 26 and 33 characters. The `test()` method is then used to check if the address matches the regular expression. Remember to adjust the regular expression based on the specific requirements of the cryptocurrency you are validating.
Nov 27, 2021 · 3 years ago
Related Tags
Hot Questions
- 86
How does cryptocurrency affect my tax return?
- 78
How can I minimize my tax liability when dealing with cryptocurrencies?
- 60
Are there any special tax rules for crypto investors?
- 40
What is the future of blockchain technology?
- 39
What are the advantages of using cryptocurrency for online transactions?
- 35
How can I buy Bitcoin with a credit card?
- 34
How can I protect my digital assets from hackers?
- 32
What are the best digital currencies to invest in right now?